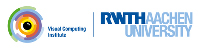 |
OpenMesh
|
45#ifndef OPENMESH_COMPILER_H
46#define OPENMESH_COMPILER_H
50#if defined(_DEBUG) || defined(DEBUG)
57#if defined(_MSC_VER) && \
58 ( defined(__ICL) || defined(__INTEL_COMPILER) || defined(__ICC) )
59# if !defined(__INTEL_COMPILER)
60# define __INTEL_COMPILER __ICL
62# define OM_USE_INTEL_COMPILER 1
70#if defined(_MSC_VER) && !defined(OM_USE_INTEL_COMPILER)
71# if (_MSC_VER == 1300)
74# define OM_OUT_OF_CLASS_TEMPLATE 0
75# define OM_PARTIAL_SPECIALIZATION 0
76# define OM_INCLUDE_TEMPLATES 1
77# elif (_MSC_VER == 1310)
80# define OM_OUT_OF_CLASS_TEMPLATE 1
81# define OM_PARTIAL_SPECIALIZATION 1
82# define OM_INCLUDE_TEMPLATES 1
83# elif (_MSC_VER >= 1400)
85# define OM_OUT_OF_CLASS_TEMPLATE 1
86# define OM_PARTIAL_SPECIALIZATION 1
87# define OM_INCLUDE_TEMPLATES 1
89# error "Version 7 (.NET 2002) or higher of the MS VC++ is required!"
92# define OM_STATIC_BUILD 1
94# define OM_REENTRANT 1
96# define OM_CC "MSVC++"
97# define OM_CC_VERSION _MSC_VER
100# if defined(__cplusplus) && !defined(_CPPRTTI)
101# error "Enable Runtime Type Information (Compiler Option /GR)!"
103# if !defined(_USE_MATH_DEFINES)
104# error "You have to define _USE_MATH_DEFINES in the compiler settings!"
107#elif defined(__BORLANDC__)
108# error "Borland Compiler are not supported yet!"
110#elif defined(__GNUC__) && !defined(__ICC)
112# define OM_GCC_VERSION (__GNUC__ * 10000 + __GNUC_MINOR__ * 100 )
113# define OM_GCC_MAJOR __GNUC__
114# define OM_GCC_MINOR __GNUC_MINOR__
115# if (OM_GCC_VERSION >= 30200)
116# define OM_TYPENAME typename
117# define OM_OUT_OF_CLASS_TEMPLATE 1
118# define OM_PARTIAL_SPECIALIZATION 1
119# define OM_INCLUDE_TEMPLATES 1
121# error "Version 3.2.0 or better of the GNU Compiler is required!"
123# if defined(_REENTRANT)
124# define OM_REENTRANT 1
127# define OM_CC_VERSION OM_GCC_VERSION
129#elif defined(__ICC) || defined(__INTEL_COMPILER)
131# define OM_TYPENAME typename
132# define OM_OUT_OF_CLASS_TEMPLATE 1
133# define OM_PARTIAL_SPECIALIZATION 1
134# define OM_INCLUDE_TEMPLATES 1
135# if defined(_REENTRANT) || defined(_MT)
136# define OM_REENTRANT 1
139# define OM_CC_VERSION __INTEL_COMPILER
141# if defined(_MSC_VER) || defined(WIN32)
142# define OM_STATIC_BUILD 1
145#elif defined(__MIPS_ISA) || defined(__mips)
153# define OM_TYPENAME typename
154# define OM_OUT_OF_CLASS_TEMPLATE 1
155# define OM_PARTIAL_SPECIALIZATION 1
156# define OM_INCLUDE_TEMPLATES 0
158# define OM_CC_VERSION _COMPILER_VERSION
161# error "You're using an unsupported compiler!"
Project OpenMesh,
© Visual Computing Institute, RWTH Aachen.
Documentation generated using
doxygen
.